1091. Shortest Path in Binary Matrix
Medium
Given an n x n
binary matrix grid
, return the length of the shortest clear path in the matrix. If there is no clear path, return -1
.
A clear path in a binary matrix is a path from the top-left cell (i.e., (0, 0)
) to the bottom-right cell (i.e., (n - 1, n - 1)
) such that:
- All the visited cells of the path are
0
. - All the adjacent cells of the path are 8-directionally connected (i.e., they are different and they share an edge or a corner).
The length of a clear path is the number of visited cells of this path.
Example 1:
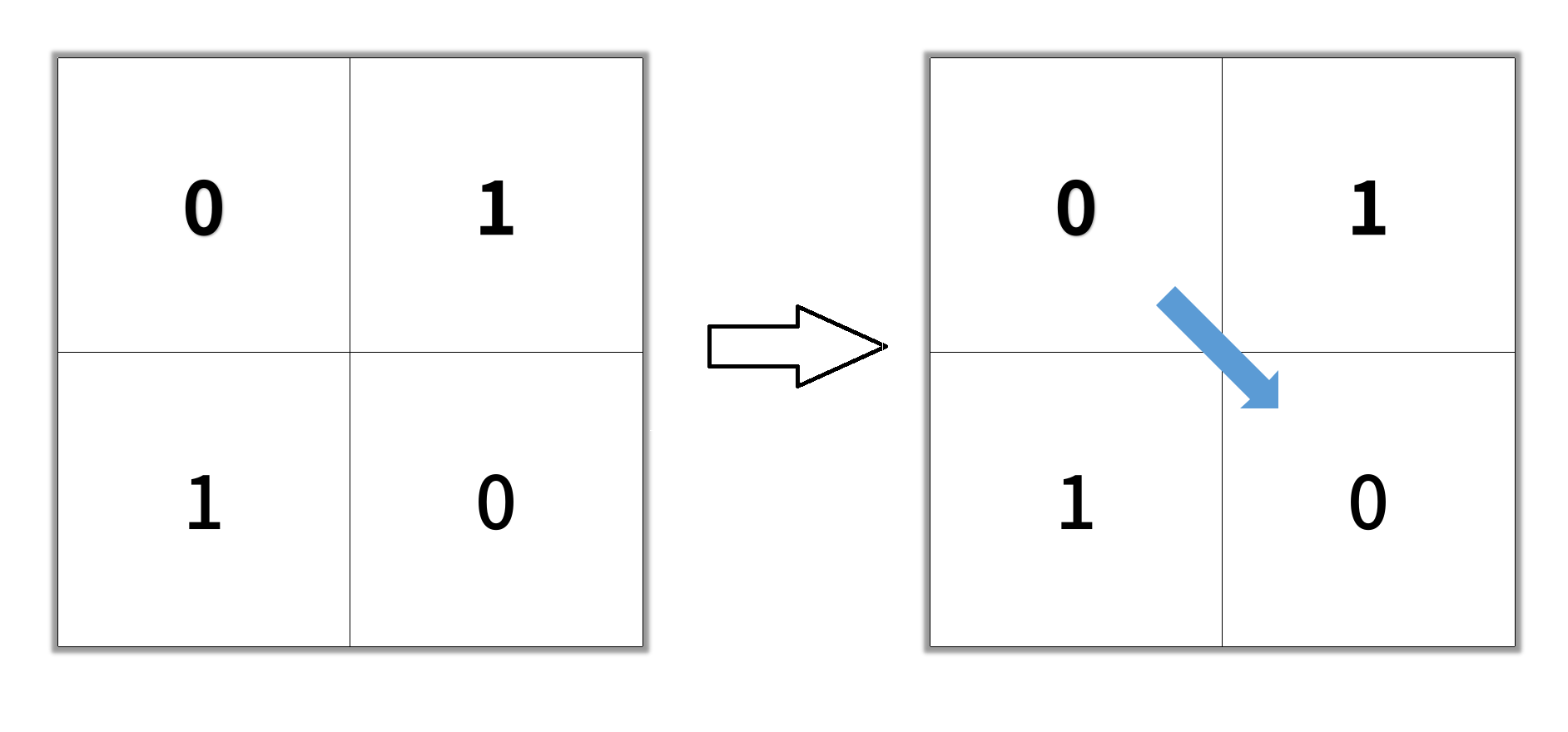
Input: grid = [[0,1],[1,0]]
Output: 2
Example 2:
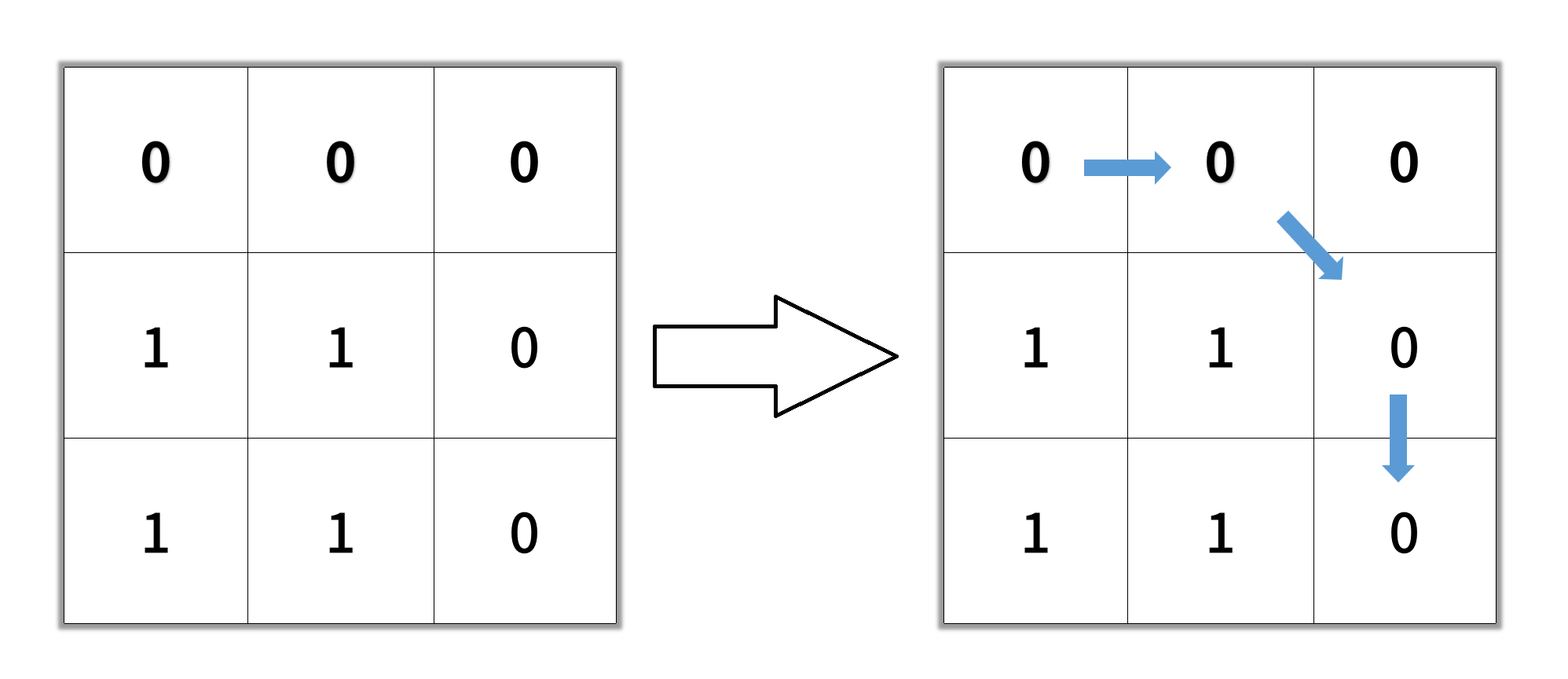
Input: grid = [[0,0,0],[1,1,0],[1,1,0]]
Output: 4
Example 3:
Input: grid = [[1,0,0],[1,1,0],[1,1,0]]
Output: -1
Constraints:
n == grid.length
n == grid[i].length
1 <= n <= 100
grid[i][j] is 0 or 1
์ด ๋ฌธ์ ๋ gird์ top-left์์ bottom-right๊น์ง์ ์ต๋จ ๊ฒฝ๋ก์ ๊ธธ์ด๋ฅผ ๊ตฌํ๋
๋ฌธ์ ์ด๊ธฐ ๋๋ฌธ์ bfs๋ฅผ ์ฌ์ฉํ์ฌ ํ์์ต๋๋ค.
/**
* @param {number[][]} grid
* @return {number}
*/
var shortestPathBinaryMatrix = function(grid) {
// ์ด๋ ๊ฐ๋ฅํ ๊ฒฝ๋ก๊ฐ ์๊ธฐ ๋๋ฌธ์ -1์ ๋ฆฌํด
if(grid[0][0] === 1) return -1
const len = grid.length
const directions = [[-1,0],[-1,1], [0,1], [1,1], [1,0], [1,-1],[0,-1],[-1,-1]]
const seen = Array.from(Array(len), ()=>Array(len).fill(false))
seen[0][0] = true
let queue = [[0,0]]
let steps = 0
// ํ์ฌ ๊ฒฝ๋ก๊ฐ grid๋ฅผ ๋ฒ์ด๋๊ฑฐ๋ 0์ด ์๋๊ฒฝ์ฐ๋ฅผ ์ฒดํฌ
const gridIsValid = (x,y) => {
return x >= 0 && x < len && y >= 0 && y < len && grid[x][y] === 0
}
while(queue.length){
const nextQueue = []
steps++
for(const [x,y] of queue){
// grid์ bottom-right์ ๋๋ฌํ์ผ๋ฏ๋ก steps ๋ฆฌํด
if(x === len-1 && y === len-1) return steps
for(const [dx,dy] of directions){
const nx = x+dx, ny = y+dy
if(gridIsValid(nx,ny) && seen[nx][ny] === false){
seen[nx][ny] = true
nextQueue.push([nx,ny])
}
}
}
queue = nextQueue
}
return -1
};
'๐ Study Note > LeetCode' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
2306. Naming a Company (0) | 2023.02.09 |
---|---|
904. Fruit Into Baskets (0) | 2023.02.07 |
2091. Removing Minimum and Maximum From Array (0) | 2023.02.01 |
209. Minimum Size Subarray Sum (0) | 2022.12.28 |
346. Moving Average from Data Stream (0) | 2022.12.26 |