2293. Min Max Game
๋์ด๋: Easy
You are given a 0-indexed integer array nums
whose length is a power of 2
.
Apply the following algorithm on nums
:
- Let
n
be the length ofnums
. Ifn == 1
, end the process. Otherwise, create a new 0-indexed integer arraynewNums
of lengthn / 2
. - For every even index
i
where0 <= i < n / 2
, assign the value ofnewNums[i]
asmin(nums[2 * i], nums[2 * i + 1])
. - For every odd index
i
where0 <= i < n / 2
, assign the value ofnewNums[i]
asmax(nums[2 * i], nums[2 * i + 1])
. - Replace the array
nums
withnewNums
. - Repeat the entire process starting from step 1.
Return the last number that remains in nums
after applying the algorithm.
Example 1:
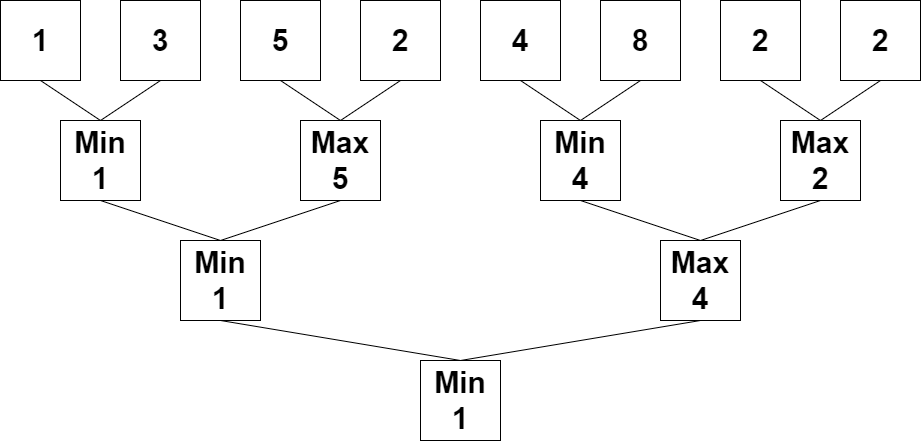
Input: nums = [1,3,5,2,4,8,2,2]
Output: 1
Explanation: The following arrays are the results of applying the algorithm repeatedly.
First: nums = [1,5,4,2]
Second: nums = [1,4]
Third: nums = [1]
1 is the last remaining number, so we return 1.
Example 2:
Input: nums = [3]
Output: 3
Explanation: 3 is already the last remaining number, so we return 3.
Constraints:
1 <= nums.length <= 1024
1 <= nums[i] <= 109
nums.length
is a power of2
.
๋ฌธ์ ์ค๋ช
๋ฐฐ์ด nums๊ฐ ์ฃผ์ด์ง ๋ nums์ ๊ธธ์ด๊ฐ 1์ด ๋ ๋๊น์ง ๋ค์ ์๊ณ ๋ฆฌ์ฆ์ ๋ฐ๋ณตํ๋ ๋ฌธ์ ์ ๋๋ค.
๋ฐฐ์ด์ ๋๊ฐ ์ฉ ๋ฌถ์ด์ ์ฐจ๋ก๋๋ก ํ์ ์ฐจ๋ก์ผ ๋ ์ต์๊ฐ์ ์ง์ ์ฐจ๋ก์ผ ๋ ์ต๋๊ฐ์ ๋ฆฌํดํ๋ค.
์ ๊ทผ๋ฒ
flag ๋ณ์๋ฅผ ์ ์ธํ์ฌ 2๊ฐ์ฉ ๋ฌถ์ ๋ฐฐ์ด์ด ํ์๋ฒ์งธ ์ธ์ง ๋๋ ์ง์ ๋ฒ์งธ์ธ์ง ์ฒดํฌํ๋ค
๊ทธ๋ฆฌ๊ณ ์ฌ๊ทํจ์๋ฅผ ํตํด nums์ ๊ธธ์ด๊ฐ 1์ด ๋ ๋๊น์ง ๋ฐ๋ณตํด์ค๋ค.
์ฝ๋
/**
* @param {number[]} nums
* @return {number}
*/
var minMaxGame = function(nums) {
if(nums.length === 1) return nums[0]
let arr = []
let flag = true
for(let i=2; i<=nums.length; i+=2){
const num = flag ? Math.min(...nums.slice(i-2,i)) : Math.max(...nums.slice(i-2,i))
flag = !flag
arr.push(num)
}
return minMaxGame(arr)
};
2294. Partition Array Such That Maximum Difference Is K
๋์ด๋: Medium
You are given an integer array nums
and an integer k
. You may partition nums
into one or more subsequences such that each element in nums
appears in exactly one of the subsequences.
Return the minimum number of subsequences needed such that the difference between the maximum and minimum values in each subsequence is at most k
.
A subsequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements.
Example 1:
Input: nums = [3,6,1,2,5], k = 2
Output: 2
Explanation:
We can partition nums into the two subsequences [3,1,2] and [6,5].
The difference between the maximum and minimum value in the first subsequence is 3 - 1 = 2.
The difference between the maximum and minimum value in the second subsequence is 6 - 5 = 1.
Since two subsequences were created, we return 2. It can be shown that 2 is the minimum number of subsequences needed.
Example 2:
Input: nums = [1,2,3], k = 1
Output: 2
Explanation:
We can partition nums into the two subsequences [1,2] and [3].
The difference between the maximum and minimum value in the first subsequence is 2 - 1 = 1.
The difference between the maximum and minimum value in the second subsequence is 3 - 3 = 0.
Since two subsequences were created, we return 2. Note that another optimal solution is to partition nums into the two subsequences [1] and [2,3].
Example 3:
Input: nums = [2,2,4,5], k = 0
Output: 3
Explanation:
We can partition nums into the three subsequences [2,2], [4], and [5].
The difference between the maximum and minimum value in the first subsequences is 2 - 2 = 0.
The difference between the maximum and minimum value in the second subsequences is 4 - 4 = 0.
The difference between the maximum and minimum value in the third subsequences is 5 - 5 = 0.
Since three subsequences were created, we return 3. It can be shown that 3 is the minimum number of subsequences needed.
Constraints:
1 <= nums.length <= 105
0 <= nums[i] <= 105
0 <= k <= 105
๋ฌธ์ ์ค๋ช
๋ฐฐ์ด nums ์ ์ต๋๊ฐ ์ต์๊ฐ ์ฐจ์ด๋ฅผ ๋ํ๋ด๋ k ๊ฐ ์ฃผ์ด์ง ๋,
์กฐ๊ฑด(์ต๋๊ฐ - ์ต์๊ฐ <= k)์ ๋ง์กฑํ๋ nums๋ฅผ ๋๋ ์ ์๋ ๊ฐ์ฅ ์์ ์๋ฅผ ๊ตฌํ๋ ๋ฌธ์ ์ด๋ค.
์ ๊ทผ๋ฒ
nums๋ฅผ ๋ด๋ฆผ ์ฐจ์ ์ ๋ ฌํ๊ณ for๋ฌธ์ ํตํด ์ฃผ์ด์ง ์กฐ๊ฑด(์ต๋๊ฐ - ์ต์๊ฐ <= k)์ ๋ง์กฑํ๋์ง ํ์ธํ๋ค.
์ฝ๋
/**
* @param {number[]} nums
* @param {number} k
* @return {number}
*/
var partitionArray = function(nums, k) {
nums.sort((a,b)=>b-a)
let maxNum = nums[0]
let count = 1
//๊ฐ์ฅ ์์๊ฐ
for(let i=1; i<nums.length; i++){
if(maxNum - nums[i] > k){
count++
maxNum = nums[i]
}
}
return count
};
2295. Replace Elements in an Array
๋์ด๋: Medium
You are given a 0-indexed array nums
that consists of n
distinct positive integers. Apply m
operations to this array, where in the ith
operation you replace the number operations[i][0]
with operations[i][1]
.
It is guaranteed that in the ith
operation:
operations[i][0]
exists innums
.operations[i][1]
does not exist innums
.
Return the array obtained after applying all the operations.
Example 1:
Input: nums = [1,2,4,6], operations = [[1,3],[4,7],[6,1]]
Output: [3,2,7,1]
Explanation: We perform the following operations on nums:
- Replace the number 1 with 3. nums becomes [3,2,4,6].
- Replace the number 4 with 7. nums becomes [3,2,7,6].
- Replace the number 6 with 1. nums becomes [3,2,7,1].
We return the final array [3,2,7,1].
Example 2:
Input: nums = [1,2], operations = [[1,3],[2,1],[3,2]]
Output: [2,1]
Explanation: We perform the following operations to nums:
- Replace the number 1 with 3. nums becomes [3,2].
- Replace the number 2 with 1. nums becomes [3,1].
- Replace the number 3 with 2. nums becomes [2,1].
We return the array [2,1].
Constraints:
n == nums.length
m == operations.length
1 <= n, m <= 105
- All the values of
nums
are distinct. operations[i].length == 2
1 <= nums[i], operations[i][0], operations[i][1] <= 106
operations[i][0]
will exist innums
when applying theith
operation.operations[i][1]
will not exist innums
when applying theith
operation.
๋ฌธ์ ์ค๋ช
nums์ operations์ด ์ ๋ ฅ๊ฐ์ผ๋ก ์ฃผ์ด์ง๋ค.
์ด๋, operations[i][0] ์ด nums์ ์กด์ฌํ๋ฉด operations [i][1]๋ก ๊ฐ์ ๋ณ๊ฒฝํ๋ ๋ฌธ์ ์ด๋ค.
์ ๊ทผ๋ฒ
๋จ์ํ๊ฒ 2์ค for๋ฌธ์ ํตํด ๋ฌธ์ ๋ฅผ ํ๊ฒ ๋๋ฉด ์๊ฐ ์ด๊ณผ๊ฐ ๋ฐ์ํ๊ธฐ ๋๋ฌธ์
nums์ operataions[i][0] ์ด ์กด์ฌํ ๊ฒฝ์ฐ ํด๋น ๊ฐ์ ์ฝ๊ฒ ๋ณ๊ฒฝํด์ฃผ๊ธฐ ์ํด
list obj์ key, value๊ฐ์ผ๋ก nums์ ๊ฐ๊ณผ ์ธ๋ฑ์ค ๋ฒํธ๋ฅผ ์ ์ฅ ํด์ค ๋ค for๋ฌธ์ ์ํํ๋ค.!
์ฝ๋
/**
* @param {number[]} nums
* @param {number[][]} operations
* @return {number[]}
*/
var arrayChange = function(nums, operations) {
const list = {}
for(let i=0; i<nums.length;i++){
list[nums[i]] = i
}
for(let i=0; i<operations.length; i++){
const idx = list[operations[i][0]] === undefined ? -1 : list[operations[i][0]]
// list์ operations[i][0]์ด ์กด์ฌํ๋ฉด
if(idx >= 0 ){
nums[idx] = operations[i][1]
delete list[operations[i][0]]
list[operations[i][1]] = idx
}
}
return nums
};
1,2,3๋ฒ ๋ชจ๋ ํ๊ณ 30๋ถ์ด ๋จ์์ง๋ง ์๊ฐ์ด ๋ถ์กฑํด์ 3 ์๋ก ๋ง๋ฌด๋ฆฌํ์ต๋๋ค.!
4๋ฒ์... ๋์์ธ ๋ฌธ์ ์๋๋ฐ ์์ง์ ์ด๋ ต๋ค์ ใ ใ ใ
๋ ์ข์ ๋ฐฉ๋ฒ ์์ผ๋ฉด ๋๊ธ๋ก ๋จ๊ฒจ ์ฃผ์ธ์~
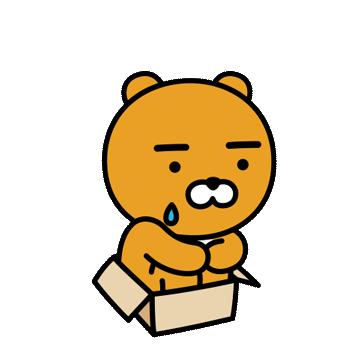
'๐ Study Note > LeetCode' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
216. Combination Sum III (0) | 2022.11.24 |
---|---|
39. Combination Sum (0) | 2022.11.24 |
51. N-Queens - ๋ฐ์ผ๋ฆฌ ๋ฌธ์ (0) | 2022.06.05 |
304. Range Sum Query 2D - Immutable - ๋ฐ์ผ๋ฆฌ ๋ฌธ์ (0) | 2022.06.03 |
867. Transpose Matrix - ๋ฐ์ผ๋ฆฌ ๋ฌธ์ (0) | 2022.06.02 |