LeetCode - Biweekly Contest 79
2283. Check if Number Has Equal Digit Count and Digit Value
난이도 : Easy
You are given a 0-indexed string num
of length n
consisting of digits.
Return true
if for every index i
in the range 0 <= i < n
, the digit i
occurs num[i]
times in num
, otherwise return false
.
Example 1:
Input: num = "1210"
Output: true
Explanation:
num[0] = '1'. The digit 0 occurs once in num.
num[1] = '2'. The digit 1 occurs twice in num.
num[2] = '1'. The digit 2 occurs once in num.
num[3] = '0'. The digit 3 occurs zero times in num.
The condition holds true for every index in "1210", so return true.
Example 2:
Input: num = "030"
Output: false
Explanation:
num[0] = '0'. The digit 0 should occur zero times, but actually occurs twice in num.
num[1] = '3'. The digit 1 should occur three times, but actually occurs zero times in num.
num[2] = '0'. The digit 2 occurs zero times in num.
The indices 0 and 1 both violate the condition, so return false.
Constraints:
n == num.length
1 <= n <= 10
num
consists of digits.
문제 설명
이 문제는 간단하게 Input값 num에서 인덱스 번호 i의 개수와 num[i]의 값이 같을 경우 true를 리턴하는 문제이다.
문제 해결법
- list 객체에 key와 value 값으로 인덱스 번호와 Input 값 num에서의 인덱스 번호의 개수를 저장해준다.
- num의 길이 만큼 for문을 순회하면서 list[i]와 num[i]를 비교하고 다를 경우 false 같으면 true를 리턴한다.!
코드
/**
* @param {string} num
* @return {boolean}
*/
var digitCount = function(num) {
const list = {}
for(let i=0; i<num.length; i++){
if(!list[i]) list[i] = 0
if(num[i] !== i) list[num[i]] = (list[num[i]] || 0) + 1
else list[i] = (list[i] || 0) + 1
}
for(let i=0; i<num.length; i++){
if(Number(num[i]) !== list[i]) return false
}
return true
};
2284. Sender With Largest Word Count
난이도 : Medium
You have a chat log of n
messages. You are given two string arrays messages
and senders
where messages[i]
is a message sent by senders[i]
.
A message is list of words that are separated by a single space with no leading or trailing spaces. The word count of a sender is the total number of words sent by the sender. Note that a sender may send more than one message.
Return the sender with the largest word count. If there is more than one sender with the largest word count, return the one with the lexicographically largest name.
Note:
- Uppercase letters come before lowercase letters in lexicographical order.
"Alice"
and"alice"
are distinct.
Example 1:
Input: messages = ["Hello userTwooo","Hi userThree","Wonderful day Alice","Nice day userThree"], senders = ["Alice","userTwo","userThree","Alice"]
Output: "Alice"
Explanation: Alice sends a total of 2 + 3 = 5 words.
userTwo sends a total of 2 words.
userThree sends a total of 3 words.
Since Alice has the largest word count, we return "Alice".
Example 2:
Input: messages = ["How is leetcode for everyone","Leetcode is useful for practice"], senders = ["Bob","Charlie"]
Output: "Charlie"
Explanation: Bob sends a total of 5 words.
Charlie sends a total of 5 words.
Since there is a tie for the largest word count, we return the sender with the lexicographically larger name, Charlie.
Constraints:
n == messages.length == senders.length
1 <= n <= 104
1 <= messages[i].length <= 100
1 <= senders[i].length <= 10
messages[i]
consists of uppercase and lowercase English letters and' '
.- All the words in
messages[i]
are separated by a single space. messages[i]
does not have leading or trailing spaces.senders[i]
consists of uppercase and lowercase English letters only.
문제 설명
Input 값으로 messages, senders가 입력될 때 message의 단어를 누가 더 많이 보냈는지 물어보는 문제이다
이때 조건으로 만약 단어를 많이 보낸 사람이 중복될 경우 이름이 사전 순서로 큰사람을 리턴한다.
문제 해결법
- list obj에 key, value 값으로 보낸 사람의 이름, message 단어 수(공백 기준 길이)를 저장한다.
- list의 key, value 값을 배열에 저장하고 단어 수를 기준으로 내림 차순 정렬해준다.
- for문을 순회하면서 중복된 단어의 수가 있는지 체크, 이때 있을 경우 이름을 사전 순으로 크기를 비교한다.
코드
/**
* @param {string[]} messages
* @param {string[]} senders
* @return {string}
*/
var largestWordCount = function(messages, senders) {
let list = {}
senders.map((name,idx)=>{
const messageLen = messages[idx].split(' ').length
list[name] = (list[name] || 0) + messageLen
})
let arr = []
for(const [key,val] of Object.entries(list)){
arr.push([key,val])
}
arr.sort((a,b)=>b[1] - a[1])
let res = arr[0]
for(let i=1; i<arr.length; i++){
if(arr[i][1] < res[1]) break;
// 이름 크기 비교
if(res[0] < arr[i][0]) res = arr[i];
}
return res[0];
};
2285. Maximum Total Importance of Roads
난이도 : Medium
You are given an integer n
denoting the number of cities in a country. The cities are numbered from 0
to n - 1
.
You are also given a 2D integer array roads
where roads[i] = [ai, bi]
denotes that there exists a bidirectional road connecting cities ai
and bi
.
You need to assign each city with an integer value from 1
to n
, where each value can only be used once. The importance of a road is then defined as the sum of the values of the two cities it connects.
Return the maximum total importance of all roads possible after assigning the values optimally.
Example 1:
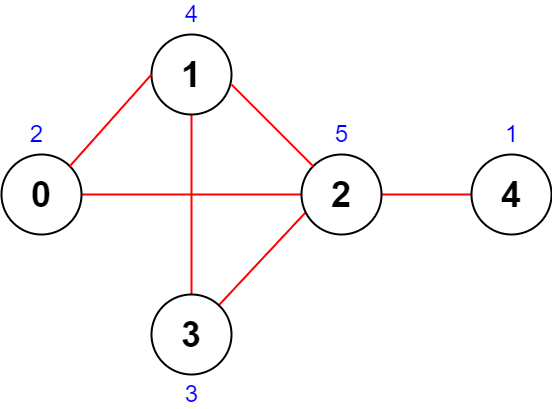
Input: n = 5, roads = [[0,1],[1,2],[2,3],[0,2],[1,3],[2,4]]
Output: 43
Explanation: The figure above shows the country and the assigned values of [2,4,5,3,1].
- The road (0,1) has an importance of 2 + 4 = 6.
- The road (1,2) has an importance of 4 + 5 = 9.
- The road (2,3) has an importance of 5 + 3 = 8.
- The road (0,2) has an importance of 2 + 5 = 7.
- The road (1,3) has an importance of 4 + 3 = 7.
- The road (2,4) has an importance of 5 + 1 = 6.
The total importance of all roads is 6 + 9 + 8 + 7 + 7 + 6 = 43.
It can be shown that we cannot obtain a greater total importance than 43.
Example 2:
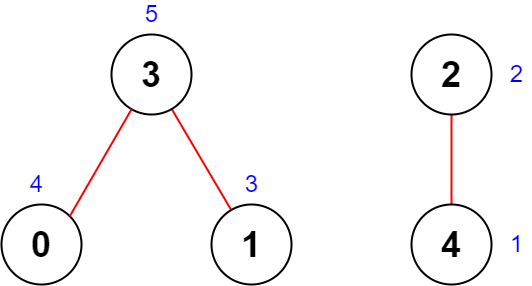
Input: n = 5, roads = [[0,3],[2,4],[1,3]]
Output: 20
Explanation: The figure above shows the country and the assigned values of [4,3,2,5,1].
- The road (0,3) has an importance of 4 + 5 = 9.
- The road (2,4) has an importance of 2 + 1 = 3.
- The road (1,3) has an importance of 3 + 5 = 8.
The total importance of all roads is 9 + 3 + 8 = 20.
It can be shown that we cannot obtain a greater total importance than 20.
Constraints:
2 <= n <= 5 * 104
1 <= roads.length <= 5 * 104
roads[i].length == 2
0 <= ai, bi <= n - 1
ai != bi
- There are no duplicate roads.
문제 설명
문제 해결법
간선 수가 많은 국가에 높은 점수(n)를 주고 list obj에 key, value 값으로 국가, 점수를 저장해준다.
그리고 roads를 순회하면서 해당 국가들의 점수를 합한 것을 리턴해준다.
코드
/**
* @param {number} n
* @param {number[][]} roads
* @return {number}
*/
var maximumImportance = function(n, roads) {
// 간선이 많은 도시에 더 높은 점수를 준다
const adjacencyList = {}
for(const road of roads){
const [x1,x2] = road
if(!adjacencyList[x1]) adjacencyList[x1] = []
if(!adjacencyList[x2]) adjacencyList[x2] = []
adjacencyList[x1].push(x2)
adjacencyList[x2].push(x1)
}
let arr = []
for(const [key,value] of Object.entries(adjacencyList)){
arr.push([key,value.length])
}
arr.sort((a,b)=>b[1] - a[1])
const valList = {}
for(const key of arr){
valList[key[0]] = n--
}
let sum = 0
for(const road of roads){
const [x1,x2] = road
sum += valList[x1]
sum += valList[x2]
}
return sum
};
후기
이번 시험은 조금 쉬웠던 거 같습니다.!! 다음에는 꼭 4 솔....🙏🙏
'LeetCode' 카테고리의 다른 글
LeetCode - Weekly Contest 296 풀이 및 후기 (0) | 2022.06.05 |
---|---|
51. N-Queens - 데일리 문제 (0) | 2022.06.05 |
304. Range Sum Query 2D - Immutable - 데일리 문제 (0) | 2022.06.03 |
867. Transpose Matrix - 데일리 문제 (0) | 2022.06.02 |
1461. Check If a String Contains All Binary Codes of Size K 문제 풀이 (0) | 2022.05.31 |