542. 01 Matrix
Medium
Given an m x n
binary matrix mat
, return the distance of the nearest 0
for each cell.
The distance between two adjacent cells is 1
.
Example 1:
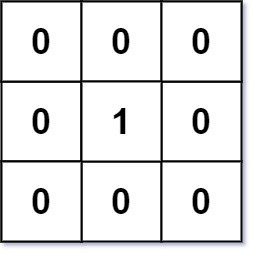
Input: mat = [[0,0,0],[0,1,0],[0,0,0]]
Output: [[0,0,0],[0,1,0],[0,0,0]]
Example 2:
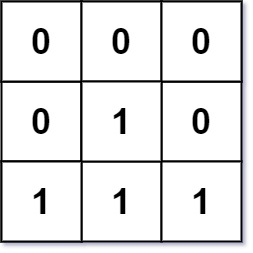
Input: mat = [[0,0,0],[0,1,0],[1,1,1]]
Output: [[0,0,0],[0,1,0],[1,2,1]]
Constraints:
m == mat.length
n == mat[i].length
1 <= m, n <= 104
1 <= m * n <= 104
mat[i][j]
is either0
or1
.- There is at least one
0
inmat
.
1์์ 0๊น์ง์ ๊ฑฐ๋ฆฌ๋ฅผ ๊ตฌํ๋ ๋ฌธ์ ์ ๋๋ค.
bfs ํ์ ๋ฐฉ์์ผ๋ก ๋ฌธ์ ๋ฅผ ํ์์ต๋๋ค.
queue์ ๋ชจ๋ 0์ ์์น ์ขํ์ ๊ฑฐ๋ฆฌ 0์ ์ด๊ธฐ์ ํธ์ํด ์ค๋๋ค.
๊ทธ๋ฆฌ๊ณ ํ์์ ํ๋ฉด์ ๋ฐฉ๋ฌธํ ๊ณณ์ด ์๋๋ผ๋ฉด ํด๋น ์ขํ์ ๊ฑฐ๋ฆฌ๋ฅผ ํ์ํด ์ค๋๋ค.
/**
* @param {number[][]} mat
* @return {number[][]}
*/
var updateMatrix = function(mat) {
let queue = []
const m=mat.length, n=mat[0].length
const directions = [[-1,0],[0,1],[1,0],[0,-1]]
const visited = Array.from({length:m},()=>Array(n).fill(false)) // ๋ฐฉ๋ฌธํ ๊ณณ ์ฒดํฌ
// ๊ทธ๋ฆฌ๋์์ ์ ํจํ์ง ์ฒดํฌ
const gridIsValid = (x,y) => {
return x>=0 && x<m && y>=0 && y<n && mat[x][y] === 1
}
// queue์ ์ขํ๋ ๊ฑฐ๋ฆฌ ํธ์
for(let row=0; row<m; row++){
for(let col=0; col<n; col++){
if(mat[row][col] === 0){
queue.push([row,col,0])
visited[row][col] = true
}
}
}
// bfs ํ์
while(queue.length){
const nextQueue = []
for(const [x,y,distance] of queue){
for(const [dx,dy] of directions){
const nx=x+dx, ny=y+dy
if(gridIsValid(nx,ny) && !visited[nx][ny]){
mat[nx][ny] = distance+1
visited[nx][ny] = true
nextQueue.push([nx,ny,distance+1])
}
}
}
queue = nextQueue
}
return mat
}
'๐ Study Note > LeetCode' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
1162. As Far from Land as Possible (0) | 2023.02.10 |
---|---|
2306. Naming a Company (0) | 2023.02.09 |
904. Fruit Into Baskets (0) | 2023.02.07 |
1091. Shortest Path in Binary Matrix (0) | 2023.02.06 |
2091. Removing Minimum and Maximum From Array (0) | 2023.02.01 |